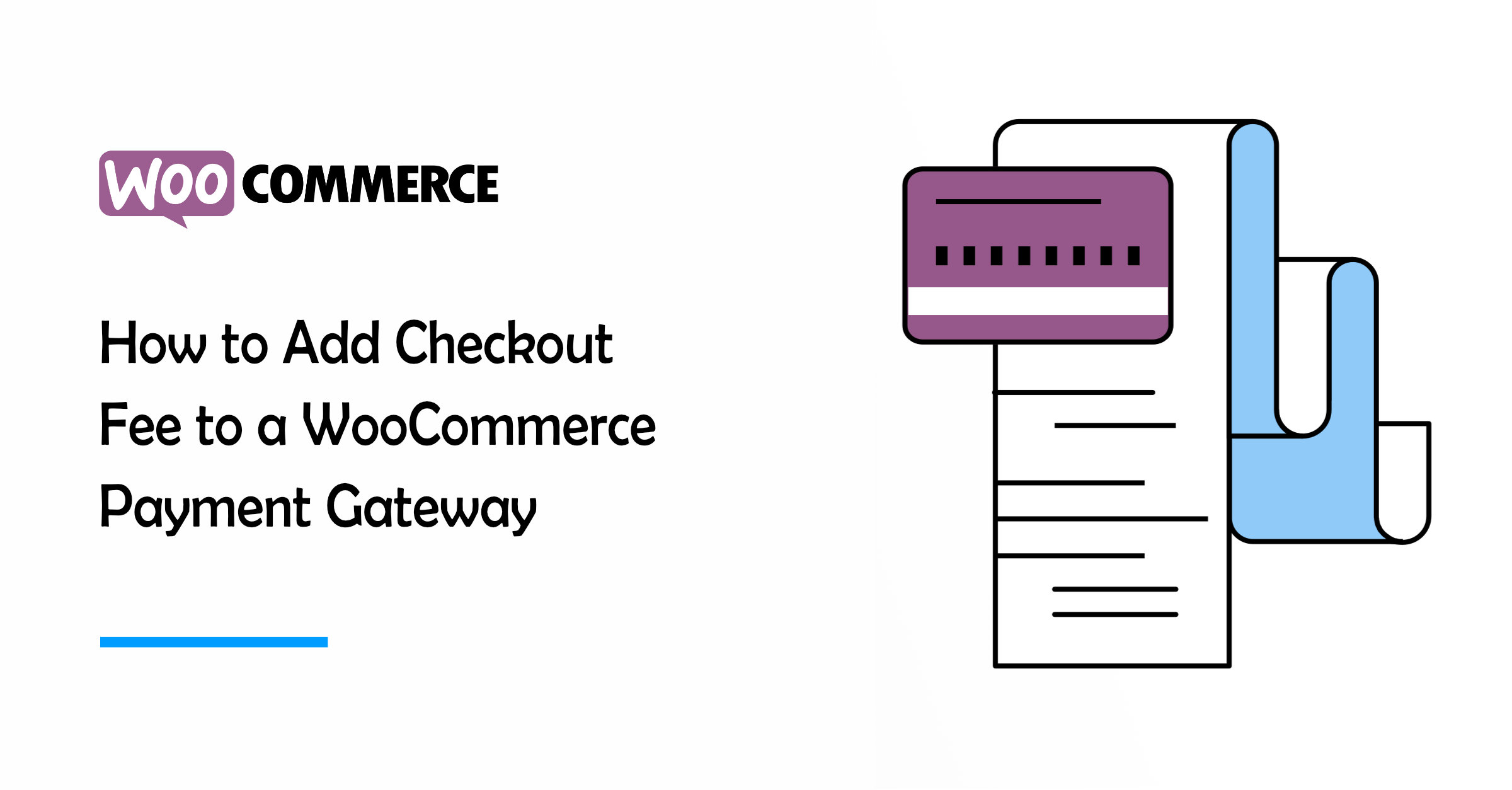
How to Add WooCommerce Checkout Fee for a Payment Gateway: Free Tutorial #1
Sometimes, it is necessary to add a Transaction or Checkout Fee on the WooCommerce Checkout page if you want to charge a specific amount from your customer as payment gateway charges. Here’s a simple PHP snippet to add a fee to the checkout for every payment or for a specific payment gateway.
Please do remember that for certain payment gateways such as PayPal, adding checkout fees is currently against their Terms of Service so make sure to check this first.
Just copy this snippet to the end of your active theme’s functions.php file or you can use the Code Snippets plugin from WordPress.org repository. Enjoy!
Snippet #1: Add Checkout Fee for all Active WooCommerce Payment Gateways
This snippet will add a fixed Transaction Charge of $5 to the Checkout page for all available action WooCommerce Payment Gateways.
/**
* @snippet WooCommerce Add fee to checkout
* @author Sayan Datta
* @testedwith WooCommerce 4.8
* @donate $10 https://paypal.me/iamsayan
*/
add_action( 'woocommerce_cart_calculate_fees', 'sayand_add_checkout_fee' );
function sayand_add_checkout_fee( $cart ) {
// Edit "Transaction Fee" and "5" below to control Label and Amount
$cart->add_fee( 'Transaction Fee', 5 );
}
Snippet #2: Add Checkout Fee to a specific WooCommerce Payment Gateway
This snippet will add a fixed Transaction Charge of $5 to the Checkout page to a specific gateway called “gateway”.
/**
* @snippet WooCommerce Add fee to checkout
* @author Sayan Datta
* @testedwith WooCommerce 4.8
* @donate $10 https://paypal.me/iamsayan
*/
// Part 1: assign fee
add_action( 'woocommerce_cart_calculate_fees', 'sayand_add_checkout_fee' );
function sayand_add_checkout_fee( $cart ) {
$chosen_gateway = WC()->session->get( 'chosen_payment_method' );
if ( $chosen_gateway == 'gateway' ) { // here gateway can be replaced by paypal, cod etc
// Edit "Transaction Fee" and "5" below to control Label and Amount
$cart->add_fee( 'Transaction Fee', 5 );
}
}
// Part 2: reload checkout on payment gateway change
add_action( 'woocommerce_review_order_before_payment', 'sayand_refresh_checkout_on_payment_methods_change' );
function sayand_refresh_checkout_on_payment_methods_change() {
?>
<script type="text/javascript">
(function($){
$( 'form.checkout' ).on( 'change', 'input[name^="payment_method"]', function() {
$('body').trigger('update_checkout');
});
})(jQuery);
</script>
<?php
}
Snippet #3: Add Checkout Fee to a specific Shipping Method and Country
This snippet will add a fixed Transaction Charge of $5 to the Checkout page to a specific shipping method called “local_pickup” and country called “US”.
/**
* @snippet WooCommerce Add fee to checkout
* @author Sayan Datta
* @testedwith WooCommerce 4.8
* @donate $10 https://paypal.me/iamsayan
*/
add_action( 'woocommerce_cart_calculate_fees', 'sayand_add_checkout_fee' );
function sayand_add_checkout_fee( $cart ) {
$chosen_methods = WC()->session->get( 'chosen_shipping_methods' );
$chosen_shipping = $chosen_methods[0];
if ( 0 === strpos( $chosen_shipping, 'local_pickup' ) && WC()->customer->get_billing_country() == 'US' ) {
// Edit "Transaction Fee" and "5" below to control Label and Amount
$cart->add_fee( 'Transaction Fee', 5 );
}
}
Does this snippet still work?
Please let me know in the comments if everything worked as expected. I would be happy to revise the snippet if you report otherwise (please provide screenshots). I have tested this code with OceanWP theme, the WooCommerce version listed above and a WordPress-friendly hosting on PHP 7.4.
It works great!
Thanks 🙂
I want to give cash back to customers opted for direct bank transfer; how to do that? Wallet plugins are not having such options. Please help.